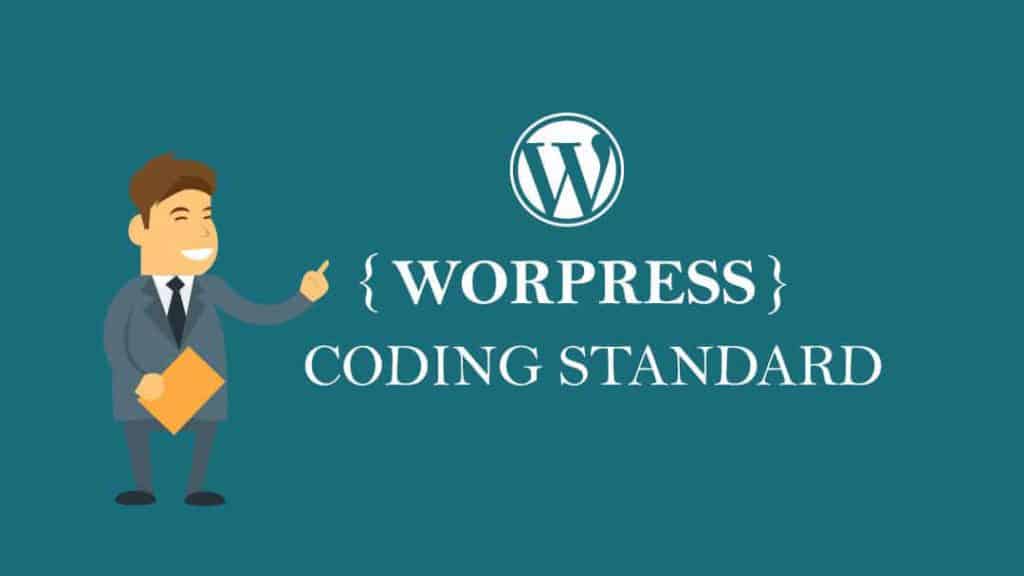
WordPress is an open-source community driven project. WordPress is very generous as it allows us to do whatever we want. Thousands of developers from all over the globe contribute to the WordPress core, plugins, and themes. To make sure that all the developers work in a harmonized way, we have the WordPress coding standards. They are a set of guidelines to make sure that code written by all developers remain clean, consistent and easily readable.
Need for Coding Standards
If you look at the code of other developers, you will find that not all of them write code the way you do. Depending on how much the code style varies, you might have a hard time figuring out what’s going on. The thing about coding styles is that no style is wrong or right. Everyone just likes to stick to their own ways as they are used to it.
Coding standards don’t tell us what is right or what is wrong. Instead, they focus on familiarity. They don’t tell us that putting braces in one place is better than putting it somewhere else. Instead, they tell us where we can expect the braces to appear.
Using standards to write high-quality code plays a key role in software development. Coding standards are the easiest way to maintain quality code.
The readability of source code directly impacts how well another developer understands the code. Coding standards improve the readability of the source code. In the process, they also make software maintenance easier.
WordPress Coding Standards
The WordPress Coding Standards are a part of the Core Contributor Handbook. The standards are split into four parts, each one dedicated to a major language used in WordPress
The guidelines are quite detailed. If you want, you can read the complete standard by following the links. Here, we are going to outline the major rules that you should try and remember. We will use the official examples from the WordPress handbook.
WordPress PHP standards
Naming Conventions
All variable and functions names must be written in lowercase. Camel case is not allowed. Words need to be separated using underscores.
function some_name( $some_variable ) { [...] }
Class names are capitalized words that are separated by underscores. If a class name is an acronym then it must be all uppercase.
class Walker_Category extends Walker { [...] }
class WP_HTTP { [...] }
Constants are written in uppercase and words are separated by underscores.
define( 'DOING_AJAX', true );
Files must be descriptively named using lowercase letters and words separated using hyphens.
my-plugin-name.php
Class file names begin with class- and all underscores are replaced with hyphens. For example, file name WP_Error becomes
class-wp-error.php
Brace Style
Braces must be used in all places, even in times when they are not needed. This means single line control structures are not allowed. The opening brace is put one the same line – not on the next.
if ( condition ) {
action1();
} elseif ( condition2 ) {
action2a();
action2b();
}
Indentation
Use indentation to reflect logical structure. Use tabs instead of spaces to enhance readability. If you find that spaces are more readable, then you can use spaces instead.
Space usage
Use white space to make the code more readable. Use spaces after commas, on both sides of logical, comparison, string and assignment operators.
For if, elseif, foreach, for, and switch, put space on both opening and closing parenthesis. Also, use space around parenthesis when calling or defining a function.
Space between parenthesis is not used when typecasting. For arrays, use space only when an index is a variable.
Line endings should be free of white space.
! foo
array( 1, 2, 3 )
$baz . '-5'
foreach ( $foo as $bar ) { ...
function my_function( $param1 = 'foo', $param2 = 'bar' ) { ..
my_function( $param1, func_param( $param2 ) );
$foo = (boolean) $bar;
$x = $foo['bar'];
$x = $foo[ $bar ];
Single and double quotes
Use single quotes when the string does not contain any variables else use double quotes.
echo '<a href="/static/link" title="Yeah yeah!">Link name</a>';
echo "<a href='$link' title='$linktitle'>$linkname</a>";
Use elseif, not else if
Stick with elseif as it provides an alternate colon syntax. Alternate colon syntax is not available for else if.
Ternary Operator
Use ternary operators to test for true conditions unless testing for a false condition makes more sense.
$musictype = ( 'jazz' == $music ) ? 'cool' : 'blah';
Yoda Conditions
Check logical statements the other way, put constants or literals on the left and variables on the right. This way if you forget an equal sign, the value won’t evaluate to true. An error will be generated instead.
if ( true == $the_force ) {
$victorious = you_will( $be );
}
WordPress HTML Coding Standards
Valid Code
All HTML code must validate against the W3C Validator. Take care as a valid code is not always good code but it’s better than invalid code.
Self-closing Elements
XHTML requires all tags closed including all self-closing tags. The official WordPress Coding Standards says that all self-closing tags should have exactly one space preceding it. Personally, I don’t follow this rule.
Attributes and Tags
Use lowercase for all attributes and tags. If the attribute is meant for humans, then do whatever that needs to be done to make it more readable.
Quotes
Provide a value for all attributes and quote them. You are free to choose between single and double quotes. Use what you like. Remember that consistency will increase readability.
Indentation
Just like PHP, use tabs to indent your code. Don’t use spaces. Make your indents reflect the logical structure. When mixing HTML and PHP code, use same indentation level for opening and closing PHP blocks.
WordPress CSS Coding Standards
Structure
- Indent CSS properties using tabs.
- Separate each section using two new lines.
- Separate each block in the section using a single line.
- Put all properties on their own line ending with a semicolon.
- Indent properties using a single tab.
- Remove the tab for the closing curly brace.
#selector-1,
#selector-2,
#selector-3 {
background: #fff;
color: #000;
}
Selectors
WordPress coding standards don’t permit the use of camel case and underscores in selectors. Use lowercase letters separated with hyphens. Human readable selectors are preferred. Use double quotes around values of attribute selectors.
#commentForm { /* Avoid camelcase. */
margin: 0;
}
#comment_form { /* Avoid underscores. */
margin: 0;
}
div#comment_form { /* Avoid over-qualification. */
margin: 0;
}
#c1-xr { /* What is a c1-xr?! Use a better name. */
margin: 0;
}
input[type=text] { /* Should be [type="text"] */
line-height: 110% /* Also doubly incorrect */
}
Properties
- Follow all properties with a colon and a white space.
- Excluding font names and vendor-specific properties, write all properties and values in lowercase.
- Use shorthand hex color codes when possible.
- Use rgba color codes when you need opacity.
- Use shorthand for styling background, margin, padding and so on.
Property Ordering
Follow any ordering that makes sense to you and is semantic in some way. The standards are permissive and don’t enforce any strict rules. Use any order you like as long as it is not random. The WordPress core chooses a logical or grouped ordering. WordPress.com themes use alphabetic ordering.
Values
- Use a space before the value.
- Always end values with a semicolon.
- 0 (zero) should not have units unless necessary.
- Include a leading zero for decimal values.
- Use space or newline to increase readability of comma separated values for one property.
- Don’t use unnecessary quotes. When needed, use double quotes instead of single quotes.
Media Queries
Use one level indent on media query rule sets. The documentation recommends placing media queries at the bottom.
Commenting
Format your comments using PHPDoc standards. Manually break long line comments at 80 characters. For highly sectioned stylesheets use a table of contents using index number 1.0, 1.1 and so on. This makes navigating the stylesheet easier.
WordPress JavaScript Coding Standards
WordPress coding standards for Javascript are based on the jQuery coding standards. Some changes were made to maintain consistency with WordPress PHP coding standards. WordPress has extensive Javascript coding standards. You can read it at this link.
Spacing
Spacing is all about readability. Use as much space as you need.
- Use tabs to indent your code.
- Remove all trailing spaces.
- Maintain a line length of 80 characters.
- Add a new line to the end of each file.
- Function body content is indented using single tab.
- Don’t use space in empty constructs (
{}
,[]
,fn())
Objects
If objects are small, then you can declare them in a single line. For long object definitions, break each property into their own line.
// Preferred
var map = {
ready: 9,
when: 4,
'you are': 15
};
// Acceptable for small objects
var map = { ready: 9, when: 4, 'you are': 15 };
// Bad
var map = { ready: 9,
when: 4, 'you are': 15 };
Semicolons
Manually put semicolons. This is a good practice instead of relying on automatic semicolon insertion.
Blocks and Curly Braces
Use braces for all conditional and loop statements. Opening braces should be placed on the same line. Closing braces are placed on a new line following the last statement on the block.
var a, b, c;
if ( myFunction() ) {
// Expressions
} else if ( ( a && b ) || c ) {
// Expressions
} else {
// Expressions
}
Chained Method Calls
For long chained methods, put each call on a new line including the first call. Use indentation to show a change in context.
elements
.addClass( 'foo' )
.children()
.html( 'hello' )
.end()
.appendTo( 'body' );
Naming Conventions
I find the WordPress Javascript naming conventions weird. They recommend the use of camel case naming for variables and functions. This is not in line with PHP.
Comments
Place a new line before comments. Use multi-line comments when placing long comments. Make the first letter of the comment capital and put a period at the end of full sentences.
Equality Checks
Consider using strict equality checks (===) in place of abstract equality checks. This rule can be neglected when checking against null or undefined.
Underscore.js
Underscore.js is a Javascript library with more than 100 utility functions baked in. This is an efficient library and WordPress recommends that you use it.
jQuery Collections
Use jQuery iterations for jQuery objects only. Use default Javascript loops to iterate over raw data.
Conclusion
Wow! That’s a whole lot of rules to follow. And of course, more rules available on the official WordPress coding standards guide. Keeping track of so many rules is very hard.
Try and follow them as best as you can. Switching midway to a different pattern can mess up big time. Remember that you don’t need to follow all of them. Just be consistent.